What is AJAX Used For
Ajax stands for Asynchronous Javascript and XML. It is a widely used script to create web pages where a user need not refresh a page when opening applications.
If you remember the early to mid ’90s, when web pages had to completely reload for a click on a menu, you get an idea of how AJAX eased things by avoiding this complete reloading.
Four important functions that AJAX does:
- Updating page without having to reload
- Request data from the server after page loads
- Retrieve information from the server after page loads
- Asynchronous sending of data in the background
The pre-requisites for learning AJAX include familiarity with HTML and JAVAScript. AJAX is based on open standards- Javascript, XML, HTML, CSS etc. It is emerging as a key component in web technology through some disadvantages such as incompatibility and maintenance issues, still, plague it.
Good examples of the use of AJAX are Google maps, Google mail.
Google Maps: Street View and Mapplets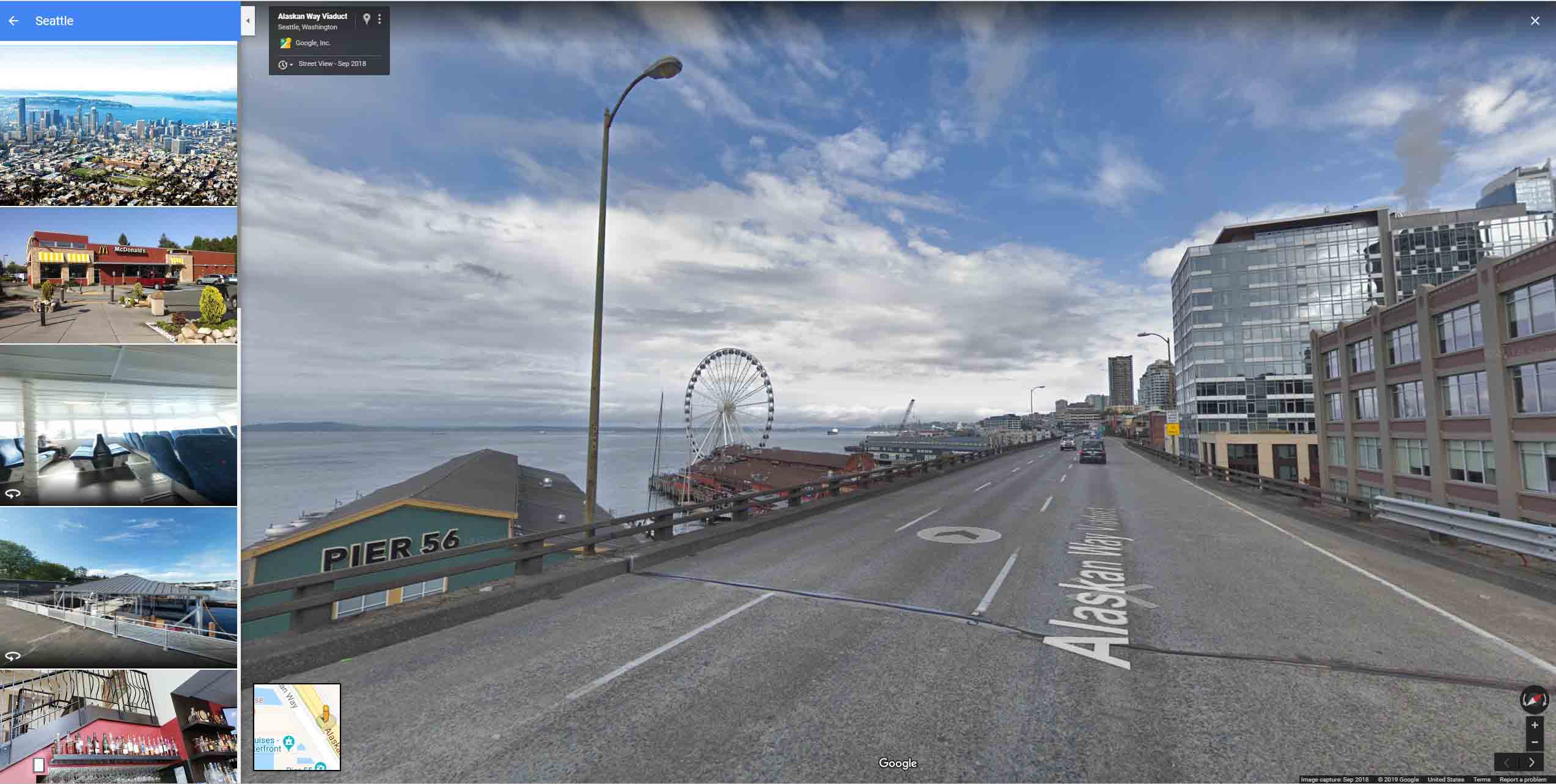
Ajaxian: When I first saw the new Street View functionality that has appeared in the Google Maps preview I was apparently impressed.
We have seen other companies taking photos of the content, but being able to walk around the Map was very cool. If I am visiting a new building, or area, I find myself checking out the area before I drive there, as it is a lot easier to locate the end point if you have seen it, and walked around the outside.
There was also a release of Mapplets, which are embeddable Google Maps mashups. You can overlay multiple mashups onto the one map, which means that you can combine the old favorites: HousingMaps.com and ChicagoCrime.org to make sure you get your new home in a suitable area!
What is exciting about these new features is that we have more tools to play with (writing Mapplets), and an excellent showcase of using Flash within an Ajax application (Maps).
A simple AJAX code is as shown:
<!DOCTYPE html>
<html>
<body>
<div id=”demo”>
<h2>AJAX can change this text</h2>
<button type=”button” onclick=”loadDoc()”>Change Content</button>
</div>
</body>
</html>
A simple AJAX program looks like the above script. Here, the first consideration is the structure. <html> tag starts the script, followed by <body> which initiates main body. As with other languages, the script ends with </body> and </html> tags.
<div> is functionality which can display information from server.
<button> is to call loadDoc() function upon clicking
loadDoc is defined outside as a function.
function loadDoc() {
var xhttp = new XMLHttpRequest();
xhttp.onreadystatechange = function() {
if (this.readyState == 4 && this.status == 200) {
document.getElementById(“demo”).innerHTML = this.responseText;
}
};
xhttp.open(“GET”, “ajax.txt”, true);
xhttp.send();
}
AJAX is a combination of XMLHttpRequest object, JAVA script, and DOM.XMLHttpRequest is a pre-defined object in most web servers such as Mozilla, Google Chrome, Apple Safari, Opera etc while HTML DOM is to display the information.DOM represents the structure of XML and HTML documents
As shown in the above script, we use this syntax to create an XMLHttpRequest object
Var xhttp = new XMLHttpRequest();
Older versions of Internet explorer (IE5 or IE6) do not have XMLHttpRequest object and use an ActiveX object instead:
variable = new ActiveXObject(“Microsoft.XMLHTTP”)
For reference:
XMLHttpRequest Object Methods
Method | Description |
new XMLHttpRequest() | Creates a new XMLHttpRequest object |
abort() | Cancelling request |
getAllResponseHeaders() | Returns header information |
getResponseHeader() | Returns specific header information |
open(method,url,async,user,psw) | Specifies the request
method: the request type GET or POST |
send() | Sending request to the server |
send(string) | Sending request to servers- particularly for POST
method |
setRequestHeader() | Adds a label/value pair to the header to be sent |
For reference:
XMLHttpRequest Object Properties
Property | Description |
onreadystatechange | If steady-state property changes defines a function to be called |
readyState | Holds the status of the XMLHttpRequest. 0: request not initialized 1:a server connected2: receipt of a request 3: processing request 4: request finished and response ready |
responseText | To return data as a string |
responseXML | To return XML data |
status | Returns the status-number of a request 200: “OK” 403: “Forbidden” 404: “Page Not Found” |
statusText | Returns the status-text |
Variable xhttp holds the value of new created XMLHttpRequest object.
While calling an XML object, both web page and XML should be on the same server.
To retrieve information from the server and send it for display, we use the following syntax:
xhttp.open(“GET”,”ajax.txt”,true)
Xttp.send()
The first command line retrieves XML file from a server. Here xhttp.open() has 3 elements. GET commands to fetch the file, the second element, a URL parameter ‘ajax.txt’ is the address of the file on the system while third element ‘true’ says the system to send data asychronously.
Instead of GET, one can use POST which is a less fast option but holds no restriction on file size.
When the variable xhttp fetches value, a ready status function can be tagged which could respond every time the ready State changes.
On ready state, change is a function that gets called when a ready state of xhttp changes.
readyState values for a variable can be:
0: request not initialized
1: a server connected
2: receipt of a request
3: processing request
4: request finished and response ready
Status function defines the status of the retrieved file:
200: “OK”
403: “Forbidden”
404: page not found
So in the above script :
if (this.readyState == 4 && this.status == 200) means that when ready state of xhttp is ‘response ready’ and file status is ‘OK’, the next line of script is executed.
Кesponse text returns the server response as a JAVA script while document.getElementById fetches specific header information from entire fetched data.
So, in the program when ‘Change content’ button is clicked, the loadDoc function gets activated. loadDoc function calls for xhttp variable which does file fetching through XMLHttpRequest and when the connection is ready and status is ok, the next text file is displayed.
The whole processing in AJAX can be summarised as follows: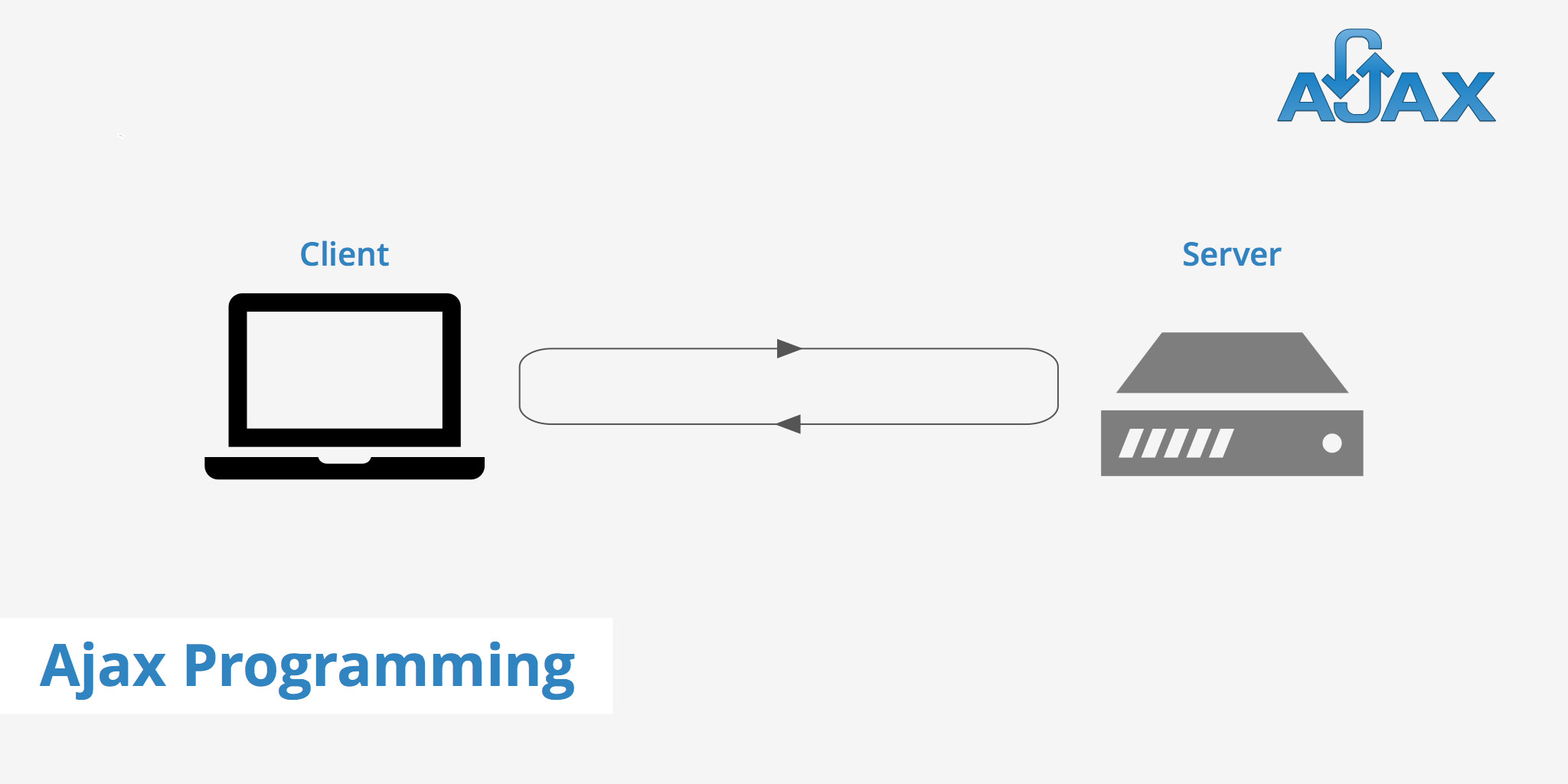
- Initial event occurs where a button is clicked
- XMLHttpRequest object is created
- Object sends a request to a web server
- Processing of request by the server
- The response is sent by a server to a web page
- JAVA script reads response
- The desired outcome is achieved
AJAX thus has many positive attributes that have derived a high interest in developers. However, there are a few issues such as the following:
- It increased the complexity while sending data from one server to the other
- AJAX-based applications are difficult to test, debug or maintain
- The immature stage of the framework supporting AJAX
- XMLHttpRequest is not yet standardised
- XMLHttpRequest is not supported in older browsers
- JAVAScript is prone to hacking
- JAVAScript has incompatibility issues
AJAX security server side has the following features:
- The security level is equivalent to regular web applications
- xml file specifies the data protection in the program
- Equally likely to be hacked like any other web application
While AJAX client side has the following features:
- JAVAScript is susceptible to hacking
- JAVAScript can lead to malfunctioning
- Downloaded JAVAScript is constrained by the sandbox security model